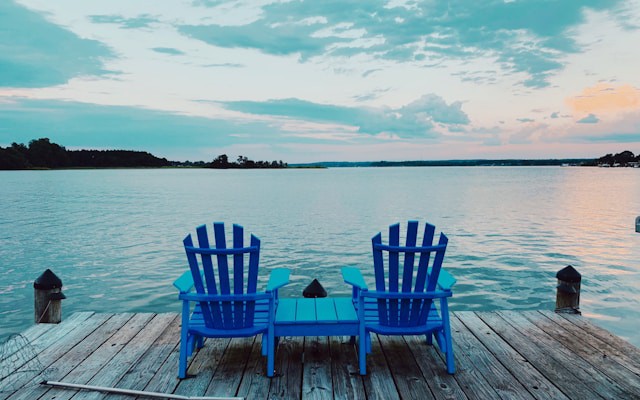
Safety is a crucial concern in cell app improvement, particularly with the rise of information breaches and cyber threats. Docker, a platform for growing, transport, and operating functions in containers, affords a number of benefits that may improve the safety of cell apps.
This text explores 5 methods Docker can enhance safety in cell app improvement, accompanied by diagrams and code snippets for a clearer understanding.
1. Isolation of Dependencies
Docker containers present a light-weight, remoted setting for operating functions. This isolation implies that every utility and its dependencies run in their very own container, lowering the danger of conflicts and vulnerabilities from shared libraries. This isolation is especially essential in cell app improvement, the place totally different functions might require totally different variations of the identical library.
In a standard improvement setting, functions share the identical working system and libraries, resulting in dependency conflicts. If one utility requires a selected model of a library that conflicts with one other, it might probably create vulnerabilities. Docker solves this by encapsulating the appliance and its dependencies in a container, guaranteeing that they function in their very own setting with out interference.
Actual-World Instance
A widely known instance of isolation advantages is Netflix, which makes use of Docker to handle its microservices structure. By isolating totally different companies, Netflix reduces the danger of 1 service affecting one other, guaranteeing a safer and dependable streaming expertise for customers.
Instance
When utilizing Docker, you’ll be able to isolate your cell app’s setting utilizing a Dockerfile:
FROM node:14
WORKDIR /app
COPY bundle.json ./
RUN npm set up
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
This Dockerfile units up a Node.js utility in an remoted setting, stopping interference from different functions or dependencies.
Diagram
┌────────────────────────┐
│ Docker Engine │
└─────────▲──────────────┘
│
┌─────────────────┴─────────────────┐
│ │
┌───────┴───────┐ ┌─────┴─────┐
│ Container A │ │Container B│
│ (Cell App 1)│ │(App 2) │
└───────────────┘ └───────────┘
2. Managed and Constant Environments
Docker permits builders to create managed and constant environments throughout totally different phases of improvement. This consistency helps in figuring out safety vulnerabilities early within the improvement cycle.
Inconsistent environments can result in discrepancies in conduct between improvement, testing, and manufacturing. These inconsistencies might cover vulnerabilities that solely floor underneath sure situations. Docker ensures that each setting — improvement, testing, and manufacturing — is similar, making it simpler to identify and repair safety points.
Actual-World Instance
Spotify makes use of Docker to handle its improvement environments. By offering constant environments, Spotify minimizes the danger of safety vulnerabilities that would come up from discrepancies between improvement and manufacturing, guaranteeing a clean person expertise throughout platforms.
Utilizing Docker Compose, you’ll be able to outline a number of companies with particular variations of dependencies, guaranteeing everybody on the workforce has the identical setting:
model: '3.8'
companies:
app:
picture: my-mobile-app:newest
ports:
- "3000:3000"
setting:
NODE_ENV: manufacturing
This configuration ensures that the appliance runs with the identical settings throughout totally different environments, lowering the prospect of introducing safety points.
3. Simplified Dependency Administration
Docker simplifies dependency administration by encapsulating all utility dependencies inside the container. This encapsulation reduces the danger of vulnerabilities from outdated libraries or parts.
Managing dependencies in conventional functions will be difficult, particularly relating to retaining libraries updated and guaranteeing compatibility. Docker means that you can specify precise variations of libraries and instruments inside your Dockerfile. This ensures that the appliance runs in a recognized state and minimizes publicity to recognized vulnerabilities.
Actual-World Instance
LinkedIn leverages Docker to handle dependencies throughout its numerous companies. LinkedIn minimizes vulnerabilities and maintains a safe platform for its customers by guaranteeing every service runs with the right variations of libraries.
With a Dockerfile, you’ll be able to specify the precise variations of dependencies:
FROM python:3.8
WORKDIR /app
COPY necessities.txt ./
RUN pip set up -r necessities.txt
COPY . .
CMD ["python", "app.py"]
This ensures that the appliance at all times runs with the required dependencies, minimizing the assault floor from vulnerabilities in third-party libraries.
4. Safe Picture Storage and Distribution
Docker supplies the power to retailer and distribute photos securely. Through the use of personal container registries, you’ll be able to make sure that solely licensed customers can entry and deploy your photos.
In lots of organizations, photos can comprise delicate data, resembling API keys, secrets and techniques, and proprietary code. Utilizing a public registry poses the danger of exposing this data. Docker permits the usage of personal registries the place entry will be tightly managed. Solely authenticated customers can pull photos, lowering the danger of unauthorized entry.
Actual-World Instance
GitHub employs Docker’s personal registries to handle container photos securely. By proscribing entry to pictures, GitHub ensures that solely licensed builders can deploy functions, minimizing the danger of information leaks and unauthorized entry.
Diagram
┌────────────────────┐
│ Non-public Registry │
│ │
│ +------------+ │
│ | Picture A | │
│ | Picture B | │
│ +------------+ │
└────────▲───────────┘
│
│
┌────────▼───────────┐
│ Docker Host │
│ │
│ +-------------+ │
│ | Container | │
│ | (App) | │
│ +-------------+ │
└────────────────────┘
Utilizing a personal registry in your Docker photos ensures that solely trusted photos are deployed to your manufacturing setting.
5. Enhanced Vulnerability Scanning
Docker integrates with numerous safety instruments that may scan container photos for recognized vulnerabilities. This proactive strategy permits builders to establish and mitigate safety dangers earlier than deployment.
With the speedy tempo of software program improvement, vulnerabilities in libraries and dependencies can emerge shortly. Docker permits the usage of automated vulnerability-scanning instruments that may examine photos for recognized vulnerabilities. This proactive safety measure permits groups to deal with vulnerabilities early, guaranteeing that solely safe photos make it to manufacturing.
Actual-World Instance
Alibaba Cloud incorporates automated vulnerability scanning in its Docker container service. They’ll tackle safety points earlier than deployment by constantly scanning photos for vulnerabilities.
You should utilize instruments like Trivy to scan your Docker photos for vulnerabilities:
trivy picture my-mobile-app:newest
This command scans the required picture and reviews any vulnerabilities discovered, permitting you to deal with them earlier than deploying.
Conclusion
Docker affords a number of vital safety advantages for cell app improvement, together with isolation of dependencies, constant environments, simplified dependency administration, safe picture storage, and enhanced vulnerability scanning. Utilizing Docker, builders can enhance their safety posture and scale back the danger of safety breaches of their cell functions.
Steps to Observe
- Implement steady integration and steady deployment (CI/CD) pipelines with Docker for automated safety checks.
- Often replace Docker photos to include the newest safety patches.
- Educate improvement groups on finest practices for safe containerization.