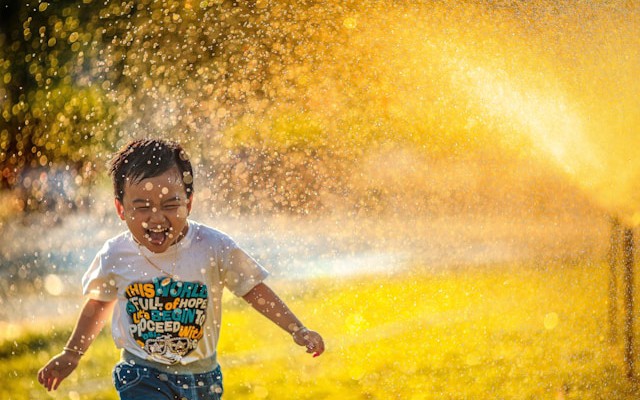
ReactJS has develop into a go-to library for constructing dynamic and responsive person interfaces. Nonetheless, as purposes develop, managing asynchronous knowledge streams turns into tougher. Enter RxJS, a strong library for reactive programming utilizing observables. RxJS operators simplify dealing with advanced asynchronous knowledge flows, making your React elements extra manageable and environment friendly.
On this article, we’ll discover RxJS operators throughout the context of ReactJS. We’ll stroll by step-by-step examples, demonstrating combine RxJS into your React purposes. By the tip of this information, you may have a strong understanding of RxJS operators and the way they’ll improve your ReactJS initiatives.
What Is RxJS?
RxJS, or Reactive Extensions for JavaScript, is a library that means that you can work with asynchronous knowledge streams utilizing observables. An observable is a set that arrives over time, enabling you to react to modifications in knowledge effectively.
However why use RxJS in ReactJS? ReactJS is inherently stateful and offers with UI rendering. Incorporating RxJS means that you can deal with advanced asynchronous operations like API calls, occasion dealing with, and state administration with higher ease and predictability.
Why Ought to You Use RxJS in ReactJS?
Improved Asynchronous Dealing with
In ReactJS, dealing with asynchronous operations like API calls or person occasions can develop into cumbersome. RxJS operators like map, filter, and debounceTime mean you can handle these operations elegantly, reworking knowledge streams as they movement by your software.
Cleaner and Extra Readable Code
RxJS promotes a purposeful programming method, making your code extra declarative. As a substitute of managing state modifications and negative effects manually, you’ll be able to leverage RxJS operators to deal with these duties concisely.
Enhanced Error Dealing with
RxJS offers highly effective error-handling mechanisms, permitting you to gracefully handle errors in your asynchronous operations. Operators like catchError and retry can robotically get better from errors with out cluttering your code with try-catch blocks.
Setting Up RxJS in a ReactJS Venture
Earlier than diving into the code, let’s arrange a fundamental ReactJS undertaking with RxJS put in.
npx create-react-app rxjs-react-example
cd rxjs-react-example
npm set up rxjs
Upon getting RxJS put in, you are prepared to start out integrating it into your React elements.
Step-by-Step Instance
Let’s stroll by an in depth instance of utilizing RxJS in a ReactJS software. We’ll create a easy app that fetches knowledge from an API and shows it in a listing. We’ll use RxJS operators to deal with the asynchronous knowledge stream effectively.
Step 1: Making a Easy React Element
First, create a brand new part referred to as DataFetcher.js
:
import React, { useEffect, useState } from 'react';
const DataFetcher = () => {
const [data, setData] = useState([]);
const [error, setError] = useState(null);
return (
{error && Error: {error}
}
{knowledge.map(merchandise => (
- {merchandise.identify}
))}
);
};
export default DataFetcher;
This part initializes state variables for knowledge and error. It renders a listing of knowledge fetched from an API and handles errors gracefully.
Step 2: Importing RxJS and Creating an Observable
Subsequent, we’ll import RxJS and create an observable for fetching knowledge. In the identical DataFetcher.js
file, modify the part to incorporate the next:
import { of, from } from 'rxjs';
import { catchError, map } from 'rxjs/operators';
import { ajax } from 'rxjs/ajax';
const fetchData = () => {
return ajax.getJSON('https://jsonplaceholder.typicode.com/customers').pipe(
map(response => response),
catchError(error => of({ error: true, message: error.message }))
);
};
Right here, we use the ajax.getJSON
methodology from RxJS to fetch knowledge from an API. The map operator transforms the response, and catchError handles any errors, returning an observable that we are able to subscribe to.
Step 3: Subscribing to the Observable in useEffect
Now, we’ll use the useEffect
hook to subscribe to the observable and replace the part state accordingly:
useEffect(() => {
const subscription = fetchData().subscribe({
subsequent: (end result) => {
if (end result.error) {
setError(end result.message);
} else {
setData(end result);
}
},
error: (err) => setError(err.message),
});
return () => subscription.unsubscribe();
}, []);
This code subscribes to the fetchData
observable. If the observable emits an error, it updates the error state; in any other case, it updates the info state. The subscription is cleaned up when the part unmounts to stop reminiscence leaks.
Step 4: Enhancing the Information Fetching Course of
Now that we’ve got a fundamental implementation, let’s improve it utilizing extra RxJS operators. For instance, we are able to add a loading state and debounce the API calls to optimize efficiency.
import {
debounceTime,
faucet
} from 'rxjs/operators';
const fetchData = () => {
return ajax.getJSON('https://jsonplaceholder.typicode.com/customers').pipe(
debounceTime(500),
faucet(() => setLoading(true)),
map(response => response),
catchError(error => of({
error: true,
message: error.message
})),
faucet(() => setLoading(false))
);
};
On this enhanced model, debounceTime
ensures that the API name is just made after 500ms of inactivity, lowering pointless requests. The faucet operator units the loading state earlier than and after the API name, offering visible suggestions to the person.
Frequent RxJS Operators and Their Utilization in ReactJS
RxJS presents a variety of operators that may be extremely helpful in ReactJS purposes. Listed here are a couple of frequent operators and the way they can be utilized:
map
The map
operator transforms every worth emitted by an observable. In ReactJS, it may be used to format knowledge earlier than rendering it within the UI.
const transformedData$ = fetchData().pipe(
map(knowledge => knowledge.map(merchandise => ({ ...merchandise, fullName: `${merchandise.identify} (${merchandise.username})` })))
);
filter
The filter
operator means that you can filter out values that do not meet sure standards. That is helpful for displaying solely related knowledge to the person.
const filteredData$ = fetchData().pipe(
filter(merchandise => merchandise.isActive)
);
debounceTime
debounceTime
delays the emission of values from an observable, making it very best for dealing with person enter occasions like search queries.
const searchInput$ = fromEvent(searchInput, 'enter').pipe(
debounceTime(300),
map(occasion => occasion.goal.worth);
);
switchMap
switchMap
is ideal for dealing with situations the place solely the newest results of an observable issues, akin to autocomplete ideas.
const autocomplete$ = searchInput$.pipe(
switchMap(question => ajax.getJSON(`/api/search?q=${question}`))
);
Superior RxJS and ReactJS Integration: Leveraging Extra Operators and Patterns
Combining Observables With merge
Generally, it is advisable deal with a number of asynchronous streams concurrently. The merge
operator means that you can mix a number of observables right into a single observable, emitting values from every as they arrive.
import {
merge,
of,
interval
} from 'rxjs';
import {
map
} from 'rxjs/operators';
const observable1 = interval(1000).pipe(map(val => `Stream 1: ${val}`));
const observable2 = interval(1500).pipe(map(val => `Stream 2: ${val}`));
const mixed$ = merge(observable1, observable2);
useEffect(() => {
const subscription = mixed$.subscribe(worth => {
console.log(worth); // Logs values from each streams as they arrive
});
return () => subscription.unsubscribe();
}, []);
In a React app, you should utilize merge to concurrently hearken to a number of occasions or API calls and deal with them in a unified method.
Actual-Time Information Streams With interval and scan
For purposes requiring real-time updates, akin to inventory tickers or stay dashboards, RxJS can create and course of streams successfully.
import { interval } from 'rxjs';
import { scan } from 'rxjs/operators';
const ticker$ = interval(1000).pipe(
scan(rely => rely + 1, 0)
);
useEffect(() => {
const subscription = ticker$.subscribe(rely => {
console.log(`Tick: ${rely}`); // Logs ticks each second
});
return () => subscription.unsubscribe();
}, []);
On this instance, scan
acts like a reducer, sustaining a cumulative state throughout emissions.
Superior Consumer Enter Dealing with With combineLatest
For advanced types or situations the place a number of enter fields work together, the combineLatest
operator is invaluable.
import {
fromEvent,
combineLatest
} from 'rxjs';
import {
map
} from 'rxjs/operators';
const emailInput = doc.getElementById('e-mail');
const passwordInput = doc.getElementById('password');
const e-mail$ = fromEvent(emailInput, 'enter').pipe(
map(occasion => occasion.goal.worth)
);
const password$ = fromEvent(passwordInput, 'enter').pipe(
map(occasion => occasion.goal.worth)
);
const kind$ = combineLatest([email$, password$]).pipe(
map(([email, password]) => ({
e-mail,
password
}))
);
useEffect(() => {
const subscription = kind$.subscribe(formData => {
console.log('Kind Information:', formData);
});
return () => subscription.unsubscribe();
}, []);
This instance listens to a number of enter fields and emits the newest values collectively, simplifying kind state administration.
Retry Logic With retryWhen and delay
In situations the place community reliability is a matter, RxJS will help implement retry mechanisms with exponential backoff.
import {
ajax
} from 'rxjs/ajax';
import {
retryWhen,
delay,
scan
} from 'rxjs/operators';
const fetchData = () => {
return ajax.getJSON('https://api.instance.com/knowledge').pipe(
retryWhen(errors =>
errors.pipe(
scan((retryCount, err) => {
if (retryCount >= 3) throw err;
return retryCount + 1;
}, 0),
delay(2000)
)
)
);
};
useEffect(() => {
const subscription = fetchData().subscribe({
subsequent: knowledge => setData(knowledge),
error: err => setError(err.message)
});
return () => subscription.unsubscribe();
}, []);
This method retries the API name as much as 3 times, with a delay between makes an attempt, bettering person expertise throughout transient failures.
Loading Indicators With startWith
To supply a seamless person expertise, you’ll be able to present a loading indicator till knowledge is offered through the use of the startWith
operator.
import {
ajax
} from 'rxjs/ajax';
import {
startWith
} from 'rxjs/operators';
const fetchData = () => {
return ajax.getJSON('https://jsonplaceholder.typicode.com/customers').pipe(
startWith([]) // Emit an empty array initially
);
};
useEffect(() => {
const subscription = fetchData().subscribe(knowledge => {
setData(knowledge);
});
return () => subscription.unsubscribe();
}, []);
This ensures the UI shows a placeholder or spinner till knowledge is loaded.
Cancelable Requests With takeUntil
Dealing with cleanup of asynchronous operations is vital, particularly for search or dynamic queries. The takeUntil
operator helps cancel observables.
import {
Topic
} from 'rxjs';
import {
ajax
} from 'rxjs/ajax';
import {
debounceTime,
switchMap,
takeUntil
} from 'rxjs/operators';
const search$ = new Topic();
const cancel$ = new Topic();
const searchObservable = search$.pipe(
debounceTime(300),
switchMap(question =>
ajax.getJSON(`https://api.instance.com/search?q=${question}`).pipe(
takeUntil(cancel$)
)
)
);
useEffect(() => {
const subscription = searchObservable.subscribe(knowledge => {
setData(knowledge);
});
return () => cancel$.subsequent(); // Cancel ongoing requests on unmount
}, []);
const handleSearch = (question) => search$.subsequent(question);
Right here, takeUntil ensures that any ongoing API calls are canceled when a brand new question is entered, or the part unmounts.
FAQs
What Is the Distinction Between RxJS and Redux?
RxJS focuses on managing asynchronous knowledge streams utilizing observables, whereas Redux is a state administration library. RxJS can be utilized with Redux to deal with advanced async logic, however they serve completely different functions.
Can I Use RxJS With Useful Elements?
Sure, RxJS works seamlessly with React’s purposeful elements. You should utilize hooks like useEffect
to subscribe to observables and handle negative effects.
Is RxJS Overkill for Small React Initiatives?
For small initiatives, RxJS may seem to be overkill. Nonetheless, as your undertaking grows and it is advisable deal with advanced asynchronous knowledge flows, RxJS can simplify your code and make it extra maintainable.
How Do I Debug RxJS in ReactJS?
Debugging RxJS code may be finished utilizing instruments just like the Redux DevTools or RxJS-specific logging operators like faucet
to examine emitted values at varied phases.
How Do I Optimize for Excessive-Frequency Occasions?
Operators like throttleTime
and auditTime
are perfect for dealing with high-frequency occasions like scrolling or resizing.
Can RxJS Change React State Administration Libraries?
RxJS just isn’t a state administration answer however can complement libraries like Redux for dealing with advanced async logic. For smaller initiatives, RxJS with BehaviorSubject
can generally substitute state administration libraries.
What Are Greatest Practices for RxJS in ReactJS?
- Use
takeUntil
for cleanup inuseEffect
to keep away from reminiscence leaks. - Keep away from overusing RxJS for easy synchronous state updates; desire React’s built-in instruments for that.
- Take a look at observables independently to make sure reliability.
Conclusion
RxJS is a strong device for managing asynchronous knowledge in ReactJS purposes. Utilizing RxJS operators, you’ll be able to write cleaner, extra environment friendly, and maintainable code. Understanding and making use of RxJS in your ReactJS initiatives will considerably improve your skill to deal with advanced asynchronous knowledge flows, making your purposes extra scalable.